Python Principles Challenges
This week I decided to test my skills and try to complete all the free challenges from python principles.
https://pythonprinciples.com/challenges/
After a lot of attempts and bug-fixing, I have solved all of them. :)
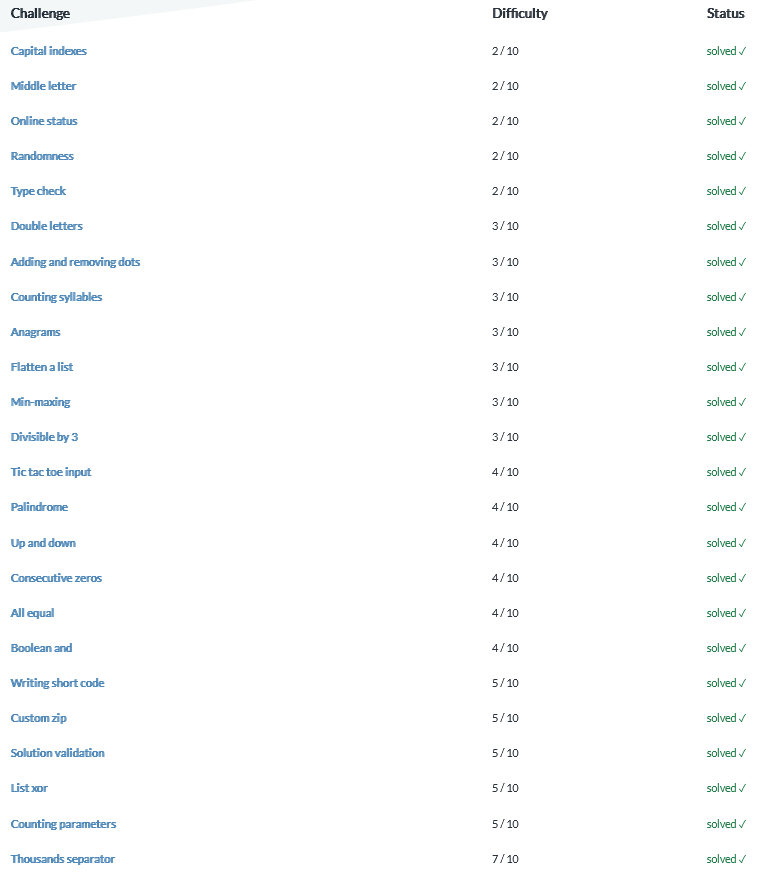
These challenges were especially rewarding as they contained new concepts that I was forced to research and learn myself. Below I showcase some of the examples I completed.
Custom zip
The built-in zip function "zips" two lists. Write your own implementation of this function.
My code:
def zap(a, b): return[(a[x], b[x]) for x in range(len(a))]
print(zap( [0, 1, 2, 3], [5, 6, 7, 8] ))
Output: [(0, 5), (1, 6), (2, 7), (3, 8)]
The function takes 2 lists and combines the corresponding items into a tuple. This was quite challenging for me as one of the conditions was - the function can only contain one line.
Thousands seperator
Write a function that converts a number to a string and adds commas as a thousands separator.
My code:
def format_number_1(num): # Function takes a single parameter 'num' string = str(num)[::-1] # Uses string slicing to reverse the number count = 0 for x in range(len(string)): # Iterates through each letter if x !=0 and (x % 3) == 0: # Conditions pass every 3 letters string = string[:x + count] + "," + string[x + count:] # adds comma to string using splits count += 1 return string[::-1] # Reverses the number again to give output
When the function is called with the number: '4591534516'
It returns: '4,591,534,516'
This was by far the hardest challenge, taking more time than all the others combined. In the end I reached a solution I am very happy with, however, I now know it can be done like this:
def format_number(num): return "{:,}".format(num)
I learnt quite a bit more doing it manually than by using the built in .format() function so I am glad I spent the time to work through it.
Leave a comment
Log in with itch.io to leave a comment.